As the world moves to working remotely and flexibly, having excellent communication platforms are pretty important. Every platform has some or other drawback, and it is not suitable for everyone. What if you could build your own chat application using the best technologies, but you don’t have to create an entire backend and infrastructure for it? Sounds interesting, right? That’s what QuickBlox does.
QuickBlox provides API integrations and Software Development Kits(SDK) for various product offerings like video calls, audio calls, messages and all those can be used in different programming languages. You can use them to create your own chat app without having to worry about the backend systems and data storage. Moreover, it also provides an option for white-labeling apps, so no one knows that you are using some third-party plugins in your application.
Angular is a javascript-based open-source web development framework developed and maintained by Google. Angular is one the most favoured Language to build web app, because of the availability of various angular js tools to create web app. Today in this article we are going to explore how to build an Angular chat app using QuickBlox SDK
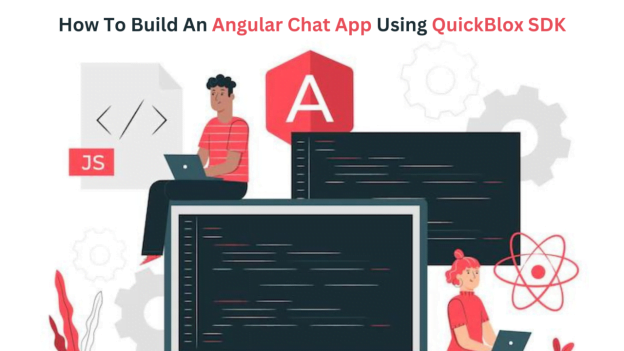
What Features are Available in QuickBlox SDK?
Real-time Chat
QuickBlox SDK leverages robust communication standards to help users connect and chat with each other in real-time over the internet by using the SDK only. It promotes faster communication so that things are not delayed. You can chat in groups or one-to-one using the real-time chat feature of this SDK. It is pretty easy to integrate into the application.
Voice and Video Calling
QuickBlox SDK offers powerful voice and video calling features. It helps you to connect with each other over internet calls, and the calls are super clear and high-quality. There is hardly any call drop, and you’ll be able to communicate in real-time at lesser costs around the world with this feature.
User Management
The SDK offers excellent APIs and functions for user management. The user management module helps find users, create their accounts, log into an existing account, reset passwords, and many other things. Using these in-built functions, you can authenticate users and allow them to use the app effectively.
You just have to pass the correct parameters to these functions, and the SDK will handle everything for you.
Data Storage
To start with the QuickBlox SDK, you don’t have to host your own storage server. There are different data storage options to choose from, and QuickBlox will provide you with storage services where you can store your app’s data. Moreover, it is agile, so you can change storage types as and when your app’s user base grows.
After knowing the features of this SDK, you might be excited to build a chat app for yourself. So let’s get into the details of building one in the next section.
How To Build An Angular Chat App Using Quickblox SDK
Setup
The first and foremost part of creating the Angular chat app using this SDK is to get the app credentials. In order to do so, follow the below steps.
- Create a new QuickBlox account by going to the official website.
- Once you have created the account, click on the new app button on the screen to create a new chat app for yourself.
- Once you click on the new app button, a new page will open where you have to configure the app type and give it a name, and get the app ready.
- If you’ve done the above step correctly, you will see your new app on the dashboard.
Head over to the overview section and save your application ID, Authorization Key, Authorization secret, and account key in a local file. These items are essential to run the app and completing the functionalities.
As we are using Angular for this, we can include the SDK in two ways, and both of them should work. One is by copy-pasting the script tag of the JS file in the base HTML file. The other one is through NPM installation, and we are going to use that way in this article.
Run the below command in your command prompt to install QuickBlox SDK.
npm install quickblox –save
Once you have installed the SDK, open a JS file and type in the below code to import and initialize the QuickBlox instance.
var QuickBlox = require('quickblox/quickblox.min');
// OR to create many QB instances
var QuickBlox = require('quickblox/quickblox.min').QuickBlox;
var QB = new QuickBlox();
var APPLICATION_ID = YOUR_APP_ID;
var AUTH_KEY = "YOUR_AUTH_KEY";
var AUTH_SECRET = "YOUR_AUTH_SECRET";
var ACCOUNT_KEY = "YOUR_ACCOUNT_KEY";
var CONFIG = { debug: true };
QB.init(APPLICATION_ID, AUTH_KEY, AUTH_SECRET, ACCOUNT_KEY, CONFIG);
In the above code, replace the keys and IDs with the ones that you copied from your app dashboard in QuickBlox. After this, we are ready to write some basic code on creating users and sending and receiving messages.
Creating Users
Creating users is the main thing that lets users use your app, and QuickBlox has easy-to-use functions for that.
First of all, you start by creating a session for the request. To do so, use the below code.
var params = { login: "username", password: "garry5santos" };
// or through email
// var params = {email: 'garry@gmail.com', password: 'garry5santos'};
QB.createSession(params, function(error, result) {
// callback function code here
});
The code will create a session for the request, and then you can proceed to do other things in the app. If a user does not have an account, you can create a user account using the below code.
var params = {
login: login,
password: "password",
full_name: "QuickBlox dummy account"
};
QB.users.create(params, function(error, result) {
if (error) {
done.fail("Create user error: " + JSON.stringify(error));
} else {
}
});
Once the code is executed, either a new account will be created for the user, or you can return the error message.
Even after the user account is created, they cannot use the app unless they have logged into the system. You can use the below code to help users log into the app.
var params = { login: "garry", password: "garry5santos" };
// or through email
// var params = {email: 'garry@gmail.com', password: 'garry5santos'};
QB.login(params, function(error, result) {
// callback function code here
});
The above code checks whether a user exists for the email and password provided in the parameters and returns an appropriate response. You can use that response to either allow users to access the app or to restrict them.
Send & Receive Messages
In QuickBlox, there are multiple dialog types, and dialogs form the basics of chats in the app. A dialog can be categorized into private, public, or group dialog. When a user sends a message, it goes to the dialog, and from there, it gets distributed based on its type.
Messages are stored in dialogs, and they are of three main types, namely text messages, Media files, and custom message types.
To send a message on a private chat, you can use the below code.
var dialog = "...";
var message = {
type: "chat",
body: "How are you today?",
extension: {
save_to_history: 1,
dialog_id: dialog._id
},
markable: 1
};
var opponentId = 78;
try {
message.id = QB.chat.send(opponentId, message);
} catch (e) {
if (e.name === 'ChatNotConnectedError') {
// not connected to chat
}
}
//...
QB.chat.onMessageListener = onMessage;
function onMessage(userId, message) {}
In the above code, you can define the message.type as chat to send a text message, and in the message.body, you have to put in the message that you are looking to send to a user. We also have to define the OpponentID as the ID to which we must send the message.
Once that is done, we use the chat.send function to send the message to the opponent.
If you’ve followed through with this, you should now have a basic working chat app. You can now design the UI of this app and add extra features by referring to the QuickBlox documentation. It is a great way to create your own app, and the SDK is being updated regularly with new features which serve most requirements, but sometimes you may need to Hire dedicated Angular Developers if you wish to create more complex and feature-rich apps.